Now that I have a leg constructed it is time for me to focus my attention on the circuit. I have decided to base my circuit design off of the
sanguino by Zack Hoeken. I made this decision for three reasons.
I want to stay close to the arduino community in order to leverage all the open source work that has been done.
There are no surface mount parts in the design, which is good because I want to keep this at the beginner level. It's also good because I want to prototype on a breadboard and SMT doesn't play nice with breadboards.
It has enough pins. I need at least 18. I want 27. The sanguino has 32.
I put in orders for all the parts I need for two sanguinos, as well as a USB to TTL cable and a
USBtinyISP bootloader last week. I was really lucky that all the orders came in last night.
First, I soldered together my USBtinyISP kit.
Then I followed
this tutorial to construct a breadboard sanguino for prototyping.
I was a novice to AVRs and the inner workings of the arduino family of boards as of last night so I was naive enough to think that it may be possible for the bootloader to magically go onto the AVR chip when you load the firmware for the first time. Nope. I got the "avrdude: stk500_getsync(): not in sync: resp=0x00" error.
Though I was naive enough to try it I was smart enough to have already made a USBtiny bootloader. However, how do you plug the USBtiny into the breadboard sanguino?
As you can imagine this was an intimidating problem! Remember, I was a novice. It's not that the information isn't provided. Technically, all you need is the schematic of the USBtinyISP and the schematic of the sanguino to figure it out. Still... I am kind of surprised that the sanguino website doesn't say much about bootloading. I mean... this is an open source project. Furthermore, arduino bootloading tutorials are hard to come by in general. This got me thinking. Why are there no / very few tutorials on bootloading? Is it to encourage people to buy their kits which have already been bootloaded? Is it because bootloading is too technical for the average arduino hobbyist to understand? Is it because no one really cares because they have no desire to design an arduino variant on their own? Maybe it's just that no one took the time. Definitively something to think about. I welcome thoughts on this.
It took me two whole hours to check and double-check my work before I felt comfortable plugging in the USBtinyISP. Here is what you need to do if you want to bootload an ATmega644P on a breadboard with a USBtinyISP.
USBtinyISP ICSP (1) goes to (b16)

USBtinyISP ICSP (2) goes to (b19)

USBtinyISP ICSP (3) goes to (b17)

USBtinyISP ICSP (4) goes to (b15)
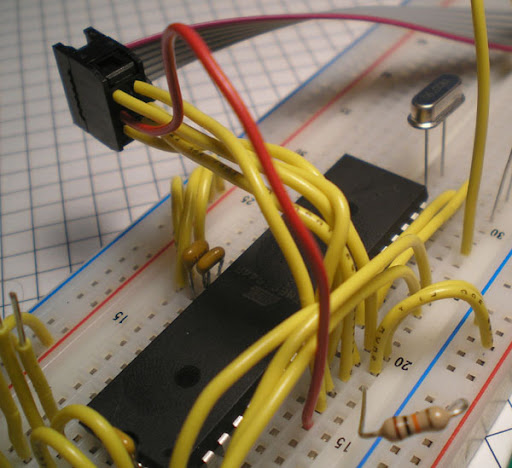
USBtinyISP ICSP (5) goes to (b18)

USBtinyISP ICSP (6) goes to (b20)
Now, assuming you have followed the tutorials on how to get your computer to recognize the USBtinyISP and you have successfully installed the
arduino app + sanguine files, the next step is to plug in your USBtinyISP. Then open up your Arduino application, select board > sanguino, select burn bootloader > USBtinyISP and wait 5 to 10 minutes. I didn't think it would take that long but I resisted the urge to unplug it and try again. It worked the very first time with patience.
I just burnt a bootloader on a fresh chip! Now I can plug in the USB to TTL cable like this:
and burn some firmware. I successfully uploaded the blink program to the ATmega644P chip before writing this.
I hope this is of use to someone.